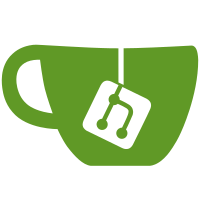
- Drastically improve performance, where non-threaded perf is now better than previous threaded perf - Process all data in-line, as opposed to building huge lists of bot data that is then processed in groups later - Memory footprint should now be relatively static no matter the amount of dumps
96 lines
2.5 KiB
C#
96 lines
2.5 KiB
C#
using Common.Models.Input;
|
|
using Common.Models.Output;
|
|
using Generator.Helpers;
|
|
using System.Diagnostics;
|
|
|
|
namespace Generator;
|
|
|
|
internal static class Program
|
|
{
|
|
internal static async Task Main(string[] args)
|
|
{
|
|
var stopwatch = Stopwatch.StartNew();
|
|
LoggingHelpers.LogToConsole("Started processing bots");
|
|
|
|
// Create list of bots we want to process
|
|
string[] botTypes = {
|
|
"assault",
|
|
"assaultgroup",
|
|
"marksman",
|
|
"pmcbot",
|
|
"exusec",
|
|
|
|
"bossbully",
|
|
"bossgluhar",
|
|
"bosskilla",
|
|
"bosskojaniy",
|
|
"bosssanitar",
|
|
"bosstagilla",
|
|
"bossknight",
|
|
"bosszryachiy",
|
|
"bossboar",
|
|
"bossboarsniper",
|
|
"bosskolontay",
|
|
"bosspartisan",
|
|
|
|
"followerbully",
|
|
"followergluharassault",
|
|
"followergluharscout",
|
|
"followergluharsecurity",
|
|
"followergluharsnipe",
|
|
"followerkojaniy",
|
|
"followersanitar",
|
|
"followerstormtrooper",
|
|
"followerbirdeye",
|
|
"followerbigpipe",
|
|
"followerzryachiy",
|
|
"followerboar",
|
|
"followerboarclose1",
|
|
"followerboarclose2",
|
|
"followerkolontayassault",
|
|
"followerkolontaysecurity",
|
|
|
|
"ravangezryachiyevent",
|
|
"peacefullzryachiyevent",
|
|
"cursedassault",
|
|
"sectantpriest",
|
|
"sectantwarrior",
|
|
"gifter",
|
|
"arenafighterevent",
|
|
"crazyassaultevent",
|
|
|
|
"shooterbtr",
|
|
|
|
"spiritspring",
|
|
"spiritwinter",
|
|
|
|
"skier",
|
|
"peacemaker",
|
|
|
|
"infectedassault",
|
|
"infectedpmc",
|
|
"infectedcivil",
|
|
"infectedlaborant",
|
|
"infectedtagilla",
|
|
};
|
|
|
|
// Read raw bot dumps and turn into c# objects
|
|
var workingPath = Directory.GetCurrentDirectory();
|
|
var dumpPath = $"{workingPath}//dumps";
|
|
List<Bot> bots = BotParser.Parse(dumpPath, botTypes.ToHashSet());
|
|
|
|
if (bots.Count == 0)
|
|
{
|
|
LoggingHelpers.LogToConsole("No bots found, unable to continue");
|
|
LoggingHelpers.LogToConsole("Check your dumps are in 'Generator\\bin\\Debug\\net6.0\\dumps' and start with 'resp.' NOT 'req.'");
|
|
return;
|
|
}
|
|
|
|
var jsonWriter = new JsonWriter(workingPath, "output");
|
|
jsonWriter.WriteJson(bots);
|
|
|
|
stopwatch.Stop();
|
|
LoggingHelpers.LogToConsole($"Finished processing bots. Took {LoggingHelpers.LogTimeTaken(stopwatch.Elapsed.TotalSeconds)} seconds");
|
|
}
|
|
}
|