mirror of
https://github.com/sp-tarkov/modules.git
synced 2025-02-13 08:10:45 -05:00
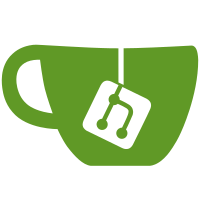
Some patches are `internal` that should be `public` so that mods can disable them when altering AI. Co-authored-by: Lacyway <20912169+Lacyway@users.noreply.github.com> Reviewed-on: SPT/Modules#152 Co-authored-by: Lacyway <lacyway@noreply.dev.sp-tarkov.com> Co-committed-by: Lacyway <lacyway@noreply.dev.sp-tarkov.com>
41 lines
1.0 KiB
C#
41 lines
1.0 KiB
C#
using SPT.Reflection.Patching;
|
|
using EFT;
|
|
using System.Reflection;
|
|
using HarmonyLib;
|
|
|
|
namespace SPT.Custom.Patches
|
|
{
|
|
/// <summary>
|
|
/// Goal: patch removes the current bot from its own enemy list - occurs when adding bots type to its enemy array in difficulty settings
|
|
/// </summary>
|
|
public class BotSelfEnemyPatch : ModulePatch
|
|
{
|
|
protected override MethodBase GetTargetMethod()
|
|
{
|
|
return AccessTools.Method(typeof(BotOwner), nameof(BotOwner.PreActivate));
|
|
}
|
|
|
|
[PatchPrefix]
|
|
private static bool PatchPrefix(BotOwner __instance, BotsGroup group)
|
|
{
|
|
IPlayer selfToRemove = null;
|
|
|
|
foreach (var enemy in group.Enemies)
|
|
{
|
|
if (enemy.Key.Id == __instance.Id)
|
|
{
|
|
selfToRemove = enemy.Key;
|
|
break;
|
|
}
|
|
}
|
|
|
|
if (selfToRemove != null)
|
|
{
|
|
group.Enemies.Remove(selfToRemove);
|
|
}
|
|
|
|
return true;
|
|
}
|
|
}
|
|
}
|